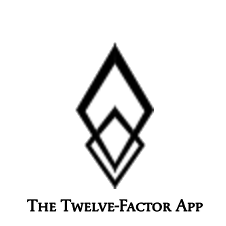
12 factor app
- Codebase - each app should have its own codebase, if there’s common code shared between multiple apps this MUST BE a library included via a dependency manager. Each app has only one codebase but multiple deploys (dev, staging, prod).
- Dependencies - dependencies must be explicitely declared and isolated, in Python for example you can use
pip
in order to manage depedencies andvirtualenv
for isolation. It is better to use a dependency manager that creates/uses lockfiles (like Poetry) since they allow repeatable and deterministic installations (you can be sure that dependencies are exactly because of the hashes being saved in the lockfile.) - Config - config and code must be separated, no costants as config. Code MUST NOT contain constant values that are used as configuration depending on the environment.
// DO NOT DO THIS
let username: string;
switch (process.env.NODE_ENV) {
case "production":
username = "produser";
break;
case "staging":
username = "staginguser";
break;
default:
throw new Error("Unknown environment", { cause: process.env.NODE_ENV });
}
// DO THIS
const username = process.env.USERNAME;
A good way to properly store configurations is to have a config.ts
file where to collect all the configuration keys.
const config = {
username: process.env.USERNAME || "defaultusername",
};
-
Backing service - a backing services include any service the app consumes over the network as part of its normal operation like databases, queue, SMTP services, caching systems, …. The app should be able to switch from a local instance of the db to a thirdy party service without the need to change the code but only by changing the configuration.
-
Build, release, run - separate strictly build, release and run operations. Each release should have a unique identifier like a timestamp
2011-04-06-20:32:17
or incrementing numberv0.0.1
-
Processes - app must be executed as a stateless process, no essential data must be stored inside memory or filesystem but rather on exetrnal stateful services like databases, this because there could be more than one instance of the app and because, even when only one process replica is up, a restart or crash could wipe all the filesystem and memory data.
-
Port binding - each app must expose itself in a self-contained way by including a web server that makes it available through a PORT, this because each app must be a backing service of another app, addressable via its config with the app’s url and port.
-
Concurrency - in order to be able to make the application scaling we need to separate its workloads into multiple processes, for instance we could divide HTTP requests (handled by a web process) and long-running background tasks by a worker process. This way, each process can be scaled indipendently from the others. You should not daemonize your app but rather execute it as a normal process whose execution is managed by the system’s process.
-
Disposability - The application must be disposable, as its processes can be started or stopped at any moment, leading to easy hot-reload during development and quick deployment in production when changing release or configuration. The startup time should be as low as possible, and every SIGTERM signal should be handled to properly close all connections with backing services after completing pending operations. This is particularly useful in modern deployment environments such as Kubernetes, where container shutdown is triggered by sending a SIGTERM signal to the container’s main process, and where the application can be replicated horizontally under heavy load conditions: the lower the startup time, the faster new requests can be handled.
-
Dev/prod parity - keep all the environments as similar as possible, this way:
- developers will be faster because they’ll spend less time in changing configuration between environments or setting up their development environments. Onboarding of new devs will be easier as well.
- both devs and ops can handle deployments and their configuration
- ther are no differences in tech stacks = no unexpected behaviours, no changes in load capabilities.
Especially in the past, when developing locally, developers used to opt for the lightweight version of a backend service (SQLite instead of PostgreSQL, for example), but nowadays most of the backends can be easily installed using modern packaging systems (homebrew, apt, …) or containerised thanks to Docker to have not only a production-like environment, but also an isolated and repeatable one.
-
Logs - application logs are the stream of aggregated, time-ordered events collected from the output streams of all running processes and backing services. The application writes logs to
stdout
, this will then collected by using log routers (Fluentd for example) and the delivered to indexing and analysis systems like Splunk, ElasticSearch or cloud-vendor solutions like Google Cloud Logging. -
Admin processes - whenever developers need to run admin processes (database migrations, running a console in order to execute arbitrary code, running one-time scripts) those need to be executed in an identical environment as the regular long-running processes of the app in order to preserve dependency isolation. They run against a release, using the same codebase and config as any process run against that release. Admin code must ship with application code to avoid synchronization issues.